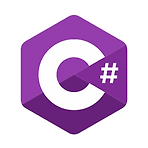
파일 스트림 (File Stream) 1. 스트림 (stream) : 파일, 네트워크 등에서 데이터를 바이트 단위로 읽고 쓰는 클래스 - Stream class는 상위 기본 클래스 -> 상속 클래스 : FileStream, MemoryStream, NetworkStream, SqlFileStream 등 - using System.IO 선언해서 사용 2. File Stream : 파일 입출력을 다루는 기본 클래스 - 상속 계층 구조 System.Object + System.MarshlByRefObject + System.IO.Stream + System.IO.FileStream - byte[ ] 배열로 데이터를 읽거나 저장 -> 형변환이 요구됨 - 파일 정보 설정에 사용 - 기본 형태 public File..
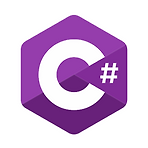
일차원 배열 - 같은 데이터형 + 변수명 + 순차적인 메모리 나열 - 참조형 : new를 통해 생성 - Array로부터 파생된 참조형 - 기본 형태 데이터형[ ] 배열명; ex) int[ ] array_name; #배열명은 참조형이기 때문에 데이터형뒤에 [ ]를 붙힘 - Array.Length 속성 - foreach 사용 가능 (읽기 전용) namespace _0614 { class Program { static void Main(string[] args) { int[] array = { 1, 2, 3, 4 }; //int[] array = new int[] { 1, 2, 3, 4 }; for (int i = 0; i < 4; i++) Console.Write("{0} ", array[i]); Conso..
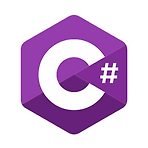
연산자 : +, -, !, ~, ++, -- 등 - ! (not)은 bool형에만 사용 ex) !-1 //0 namespace _0613 { class Program { static void Main(string[] args) { bool bFlag = false; Console.WriteLine("{0} {1} {2}", !bFlag, !true, !false); //true false true } } } 산술 연산자 : *, /, %, -, + - string에서 +는 문자열 연결 - 정수/부동 + "문자열" = "문자열" namespace _0613 { class Program { static void Main(string[] args) { string str = "3" + ".14"; Consol..
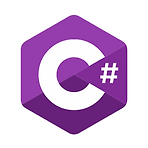
값 형식 - System.Object + System.ValueType 에서 파생 - 변수가 직접 값을 저장하는 형 - 기본 데이터형 - 구조체 - 열거형 - 선언 vs 생성 (new) namespace _0612 { class Program { static void Main(String[] args) { int val1 = 12; int val2 = new int(); //초기화를 하지 않아도 0 값이 지정되어있음 //Object 객체를 통해서 상속을 받고 있기 때문 Console.WriteLine("{0} {1}", val1, val2); //12 0 } } } 참조 형식 - 한 객체를 참조형 변수를 사용할 때, 참조형에 의해 내용이 바뀌면 객체에 영향을 줌 - class, interface, del..
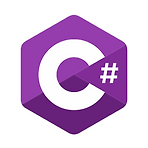
사용자 지정형 1. struct - 기본 형태 public struct 구조체명 { //멤버, 속성, 매소드 } namespace _0612 { public struct MyStruct { public const float PI = 3.14f; public static int Age = 12; } class Program { static void Main(string[] args) { Console.WriteLine("{0} {1}", MyStruct.PI, MyStruct.Age); } } } namespace _0612 { public struct MyStruct { public int Age; //생성자 - 생성과 동시에 초기화 public MyStruct(int inAge) { Age = inAg..
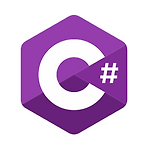
표준 입력 - Console.Readkey( ) : 사용자가 눌린 키 한 문자 정보를 리턴하는 메소드 - 함수 원형 : 오버로딩 public static ConsoleKeyInfo ReadKey( ) public static ConsoleKeyInfo ReadKey(bool intercept) : true이면 화면 출력을 하지 않고, false이면 화면 출력 - ConsoleKeyInfo : 키의 문자와 shift, alt, ctrl 보조키 상태 포함 - ConsoleKeyInfo 속성 : ConsolekeyInfo.Key, ConsoleKeyInfo.KeyChar, ConsoleKey.A, ConsoleKey.Escape 등 namespace _0612 { class Program { static vo..
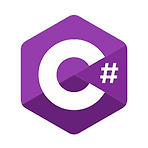
데이터 변환 - ToString( ) : 현재 값을 문자열로 변환 - Parse( ) : 문자열 원래 데이터형으로 변환 - Convert.ToXXX( ) namespace _0612 { class Program { static void Main(string[] args) { int value1 = 127; string str1 = value1.ToString(); //int를 문자열로 변환 Console.WriteLine(str1); int value2 = Convert.ToInt32(str1); // string을 int로 변환 Console.WriteLine(value2); string str2 = "3.14"; float value3 = float.Parse(str2); //문자열을 float로 변..
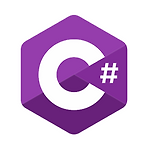
기본 데이터형 - object로부터 파생된 객체 ex) System.Object == object; - CTS에서 정의된 객체 형태 CTS byte 정수형 bool System.Boolean 1 byte char System.Char 2 byte byte System.Byte 1 byte sbyte System.SByte 1 byte short System.Int16 2 byte ushort System.UInt16 2 byte int System.Int32 4 byte uint System.UInt32 4 byte long System.Int64 8 byte ulong System.UInt64 8 byte #s (signed) : 음의 부호 #u (unsigned) : 양의 부호 실수형 float Sy..
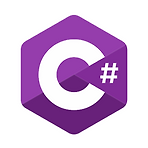
.NET FRAMEWORK 구성요소 1. Class 라이브러리 - C# 언어 + 클래스 라이브러리 = C# 프로그래밍 - MSDN 참고 (http://msdn.Microsoft.com) - BCL (Basic Class Library) : 기본 클래스 - Window Form : 윈도우 응용 프로그램 제작을 위한 클래스 라이브러리 - ASP.NET : 웹 클래스 라이브러리 - ADO.NET : 데이터베이스 클래스 라이브러리 2. CLR (Common Language Runtime) - 역할 : 컴파일된 C# 코드를 실행 - MISL, IL(Intermediate Language) - 중간언어 - Visual Studio에서 C# 코드를 컴파일한 코드 - *.exe / *.dll - .NET 언어인 VB,..