티스토리 뷰
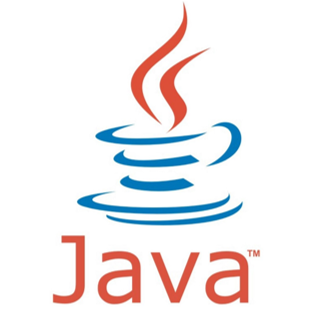
import java.util.Scanner;
abstract class Memory {
protected int[] m;
protected int count;
Memory(){ //생성자
m = new int[20];
count = 0; //초기화
}
public void push(int i) {
if(full()) {
m[count++] = i;
}
}
//추상 클래스
abstract int pop();
abstract boolean full();
abstract boolean empty();
}
class MyStack extends Memory{
@Override
public int pop() {
if(empty()) {
return m[--count];
}
else
return -1;
}
@Override
public boolean full() {
if(count == 20) {
System.out.println("FULL");
return false;
}
else
return true;
}
@Override
public boolean empty(){
if(count == 0) {
System.out.println("EMPTY");
count = 0;
return false;
}
else
return true;
}
}
class MyQueue extends Memory{
private int front;
public MyQueue() {
front = 0;
}
@Override
public int pop() {
if(empty()) {
return m[front];
}
else
return -1;
}
@Override
public boolean full() {
if(count == 20 && front == 0) {
System.out.println("FULL");
return false;
}
return true;
}
@Override
public boolean empty() {
if(count == front) {
System.out.println("EMPTY");
count = front = 0;
return false;
}
else
return true;
}
}
public class SQ {
public static void main(String[] args) {
Memory m = null;
MyStack ms = new MyStack();
MyQueue mq = new MyQueue();
Scanner s = new Scanner(System.in);
int c = 0;
while(c!=3) {
System.out.println("1.Stack 2.Queue");
System.out.print("선택 : ");
c = s.nextInt();
switch(c) {
case 1 : //stack
m = ms;
System.out.println("1.Push 2.Pop");
System.out.print("선택 : ");
int sc = s.nextInt();
if(sc == 1) {
System.out.print("입력 : ");
m.push(s.nextInt());
}
if(sc == 2) {
System.out.println(m.pop());
}
break;
case 2 : //queue
m = mq;
System.out.println("1.Push 2.Pop");
System.out.print("선택 : ");
int qc = s.nextInt();
if(qc == 1) {
System.out.print("입력 : ");
m.push(s.nextInt());
}
if(qc == 2) {
System.out.println(m.pop());
}
break;
}
}
s.close();
}
}
'LANGUAGE > JAVA' 카테고리의 다른 글
[JAVA] INNER CLASS - 내부 클래스 (0) | 2018.03.22 |
---|---|
[JAVA] INTERFACE - 인터페이스 (0) | 2018.03.22 |
[JAVA] CLASS - 클래스 (0) | 2018.03.21 |
[JAVA] 문자열 계산기 (0) | 2018.03.21 |
[JAVA] 성적 처리 (0) | 2018.03.20 |
댓글